Thank God—that’s the last time I’m going to type that word for awhile. The meme is dead, long live the SDK.
As a way to celebrate the lifting of the NDA, we bring you some very special source code. To wile away the time between our product submission and the launch of the App Store, my buddy Anthony Piraino and I worked on this very special treat. Something that will be familiar to all developers who have had to keep their mouths shut since March 6th, 2008. Just compile the source code and install it on your device. Typing pleasures await.
(You’ll need to install Twitterrific from the App Store to get the full user experience. But you’ve done that already, right?)
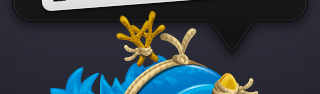
Besides being a fun inside joke, this very special application also shows an important aspect of iPhone development: URL schemes.
As we’ve seen many other times, the needs of a mobile user are very different than those of a desktop user. On the desktop, tight integration of several application domains makes applications like Coda a joy to use.
On the phone it’s better to focus on one task. From what I’ve seen, the best iPhone applications do one thing and do it well. Supporting URL schemes in your application makes that single task more attractive to other developers and users. It leads to what my friend Daniel Jalkut has aptly called the “Un-Coda-fication” of iPhone apps.
The benefit for a developer is obvious: it minimizes the scope of an application and the attendant memory footprint. You could write your own Twitter update code using a NSURLConnection, or you could use one line of code like this:
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:@"twitterrific:///post?message=EASY"]];
There is a less obvious, but equally important benefit when you look at URL schemes from a user’s point-of-view. Since your application’s scope is limited to one task, users will depend on it when they want to perform that task. Even if that task is in the context of another application.
An example of this is sharing photos. Users know that the Camera application takes pictures and that the Mail application sends messages. You don’t see a camera button in Mail; you see an “Email Photo” button in your Camera Roll. The user’s first task it to take a picture and the next task is to mail it.
Since we’re not Apple, we can’t achieve the high level of integration between the Camera Roll and the Mail application. But we can use URL schemes to accomplish much the same thing.
I worked with Fraser Speirs and Ian Baird during the development of Exposure and Cocktails so that their applications could support a “Post to Twitter” button. Clicking on that button initiates a workflow that lets the user share what they’re looking at on Flickr or what kind of drink they’re enjoying. Leaving their app/task and launching Twitterrific makes complete sense.
If you’d like to include a “Post to Twitter” button in your own application, all the code you need is in the postToTwitter: method in the very special application mentioned above. If you want to handle your own URL scheme, take a look at application:handleOpenURL: in the application delegate. Be careful about validating inputs: you don’t want malicious URLs to do bad things.
Not to dampen your enthusiasm, but please be aware of a couple of limitations with URL schemes. First, there is no way to know if a URL scheme is supported or not (rdar://problem/5726829). Currently, the best you can do is to performSelector:withObject:afterDelay: then openURL:. If the selector gets called you know that the URL failed to open. Also, be aware that deleting an application can sometimes leave the URL registration database in a state where it no longer recognizes a scheme (rdar://problem/6045562). This only happens when two applications support the same URL scheme, so you can avoid the problem by using a unique scheme name. Please use the Radar bug ID# for a “me too” bug report if this becomes a problem in your own application.
Now let’s enjoy our newfound freedom to discuss the iPhone SDK and the first of many sample code releases on this site!
Update October 1st, 2008: As Jonathan Rentzsch and Thomas Ptacek point out, URL schemes can be an attack vector for your application. Pay particular attention to the code in -application:handleOpenURL: in your application delegate. If you find any vulnerabilities in my code, please let me know so I can update this essay. Thanks!
Update October 7th, 2008: Once your application supports URL schemes, it’s likely that you’ll want to provide a bookmarklet in Safari. Here’s the one we use in Twitterrific:
javascript:window.location='twitterrific:///post?message='+escape(window.location)
The hardest part about doing this is guiding the user through the setup process. Joe Maller came up with a simple solution that lets the user get the Javascript into their bookmark list. This was later refined by Alexander Griekspoor. Make sure to view the source on these pages for additional hints and installation instructions.
If you agree that this setup process is too difficult for end users, please submit a duplicate bug for Radar ID# 5935641. Thanks!
Update February 4th, 2012: UIApplication now provides a -canOpenURL: method that lets you check if there is an application installed that supports the URL scheme.